Move or Interpolate Over Time in Unity
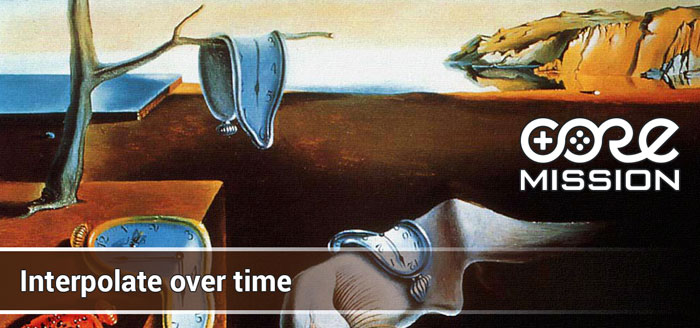
It is very frequent question on forums: “How to move or interpolate over time in Unity?”.
If you don’t use any tweener plugins, you can easily do it calculating delta for Lerp using time passed from start.
Like this, for example:
using System; using System.Collections; using UnityEngine; public class MoveController : MonoBehaviour { private RectTransform rectTransform; // Use this for initialization void Start () { rectTransform = GetComponent<RectTransform>(); } // Update is called once per frame void Update () { if (Input.GetKeyDown(KeyCode.Z)) { StartCoroutine(DoMove(3f, Vector2.zero)); } } private IEnumerator DoMove(float time, Vector2 targetPosition) { Vector2 startPosition = rectTransform.anchoredPosition; float startTime = Time.realtimeSinceStartup; float fraction = 0f; while(fraction < 1f) { fraction = Mathf.Clamp01((Time.realtimeSinceStartup - startTime) / time); rectTransform.anchoredPosition = Vector2.Lerp(startPosition, targetPosition, fraction); yield return null; } } }
This script moves object to the center of container for 3 seconds. It is easily can be adapted to rotate over time, or to move object in 3D over time, etc.
Did you like the article? Share with friends: